Error: Call to a Member Function GetCollectionParentID() on Null
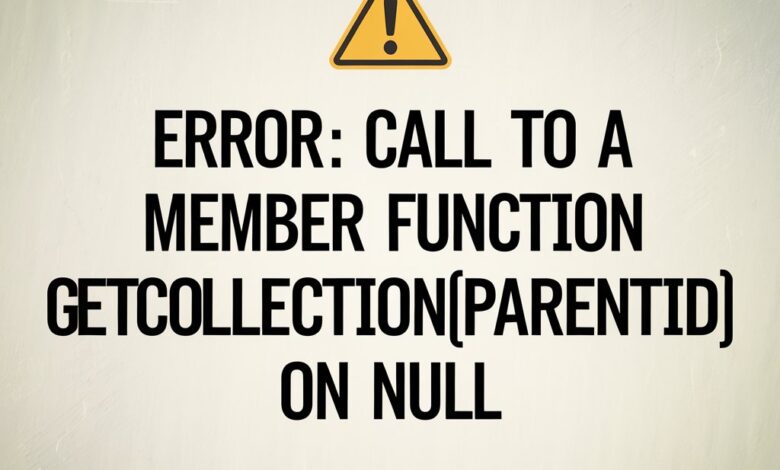
When encountering the error “Call to a member function getCollectionParentID() on null,” developers often find themselves puzzled. This error typically emerges in PHP-based applications, such as those built with content management systems like ConcreteCMS. Understanding the underlying causes and resolving this issue is crucial for maintaining application stability and functionality.
Table of Contents
What Does This Error Mean?
The error message indicates that a script is call to a member function getcollectionparentid() on null function on a variable that is null. In simpler terms, the object required for the function call does not exist at the moment the function is executed. As a result, PHP throws a fatal error, halting the execution of the script.
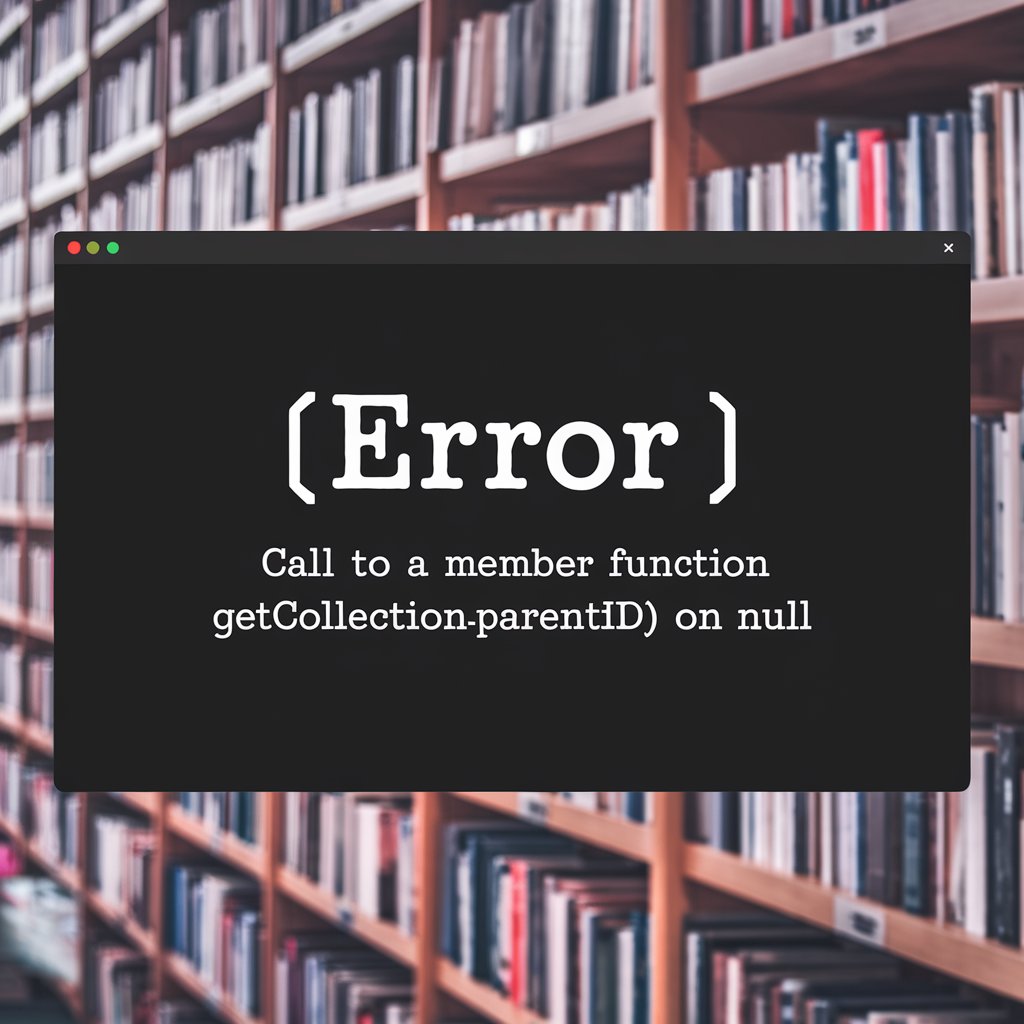
Common Scenarios That Trigger This Error
This error can arise in several scenarios, often due to improper handling of data or misconfigured code. Below are some of the most common situations:
- Missing Object Initialization: The object on which
getCollectionParentID()
is called hasn’t been initialized properly. - Database Issues: The queried data is missing or the record doesn’t exist in the database.
- Routing Problems: In content management systems, incorrect routing can result in an object being null.
- Coding Errors: Typos, logical mistakes, or failure to check for null values before invoking methods can also cause this issue.
How to Diagnose the Error
Identifying the root cause of the “Call to a member function getCollectionParentID() on null” error involves a systematic approach. Here are the key steps to diagnose:
- Check the Stack Trace: Review the stack trace provided in the error log to pinpoint where the issue occurred.
- Inspect Object Initialization: Ensure that the object being used is initialized before calling its methods.
- Verify Database Records: Confirm that the data required by the application exists in the database.
- Debug with Logging: Insert debug statements to log the state of variables leading up to the error.
Resolving the Error
The solution to this error depends on the context in which it arises. Below are actionable steps to fix the issue:
- Initialize the Object: Ensure the object is instantiated properly. For example:
$collection = Page::getByID($pageID);
if ($collection) {
$parentID = $collection->getCollectionParentID();
} else {
// Handle null scenario
echo "Page not found.";
}
- Add Null Checks: Always verify that an object is not null before invoking its methods. This can be achieved using conditional statements.
- Handle Missing Data Gracefully: If the error stems from missing database entries, ensure your application can handle such cases. For example:
if (!$pageID) {
echo "Invalid page ID.";
}
- Fix Routing Issues: If the error occurs due to incorrect routing, update the route configuration to ensure the correct object is retrieved.
- Implement Error Logging: Use PHP’s error logging features to monitor issues in real-time. For example:
error_log("Object is null in file " . __FILE__ . " on line " . __LINE__);
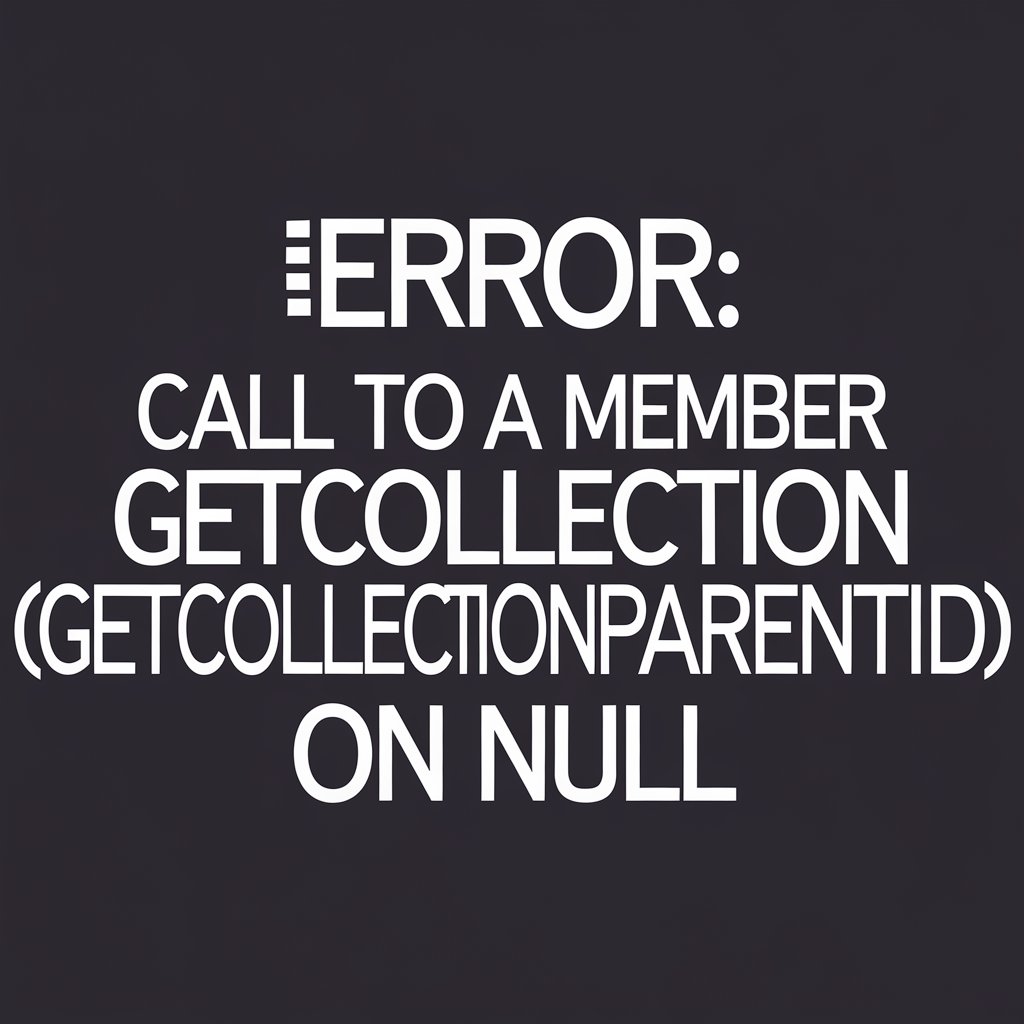
Preventing Similar Errors in the Future
To avoid encountering the “Call to a member function getCollectionParentID() on null” error in the future, adhere to the following best practices:
- Adopt Defensive Coding: Always validate input and check object states before accessing methods or properties.
- Use Exception Handling: Wrap critical code sections in try-catch blocks to handle unexpected issues gracefully.
- Follow Coding Standards: Maintain a consistent coding style and use tools like static analysis to catch potential issues early.
- Test Thoroughly: Regularly test your application in various scenarios to ensure edge cases are handled.
- Keep Systems Updated: Ensure your CMS, frameworks, and libraries are up-to-date to benefit from bug fixes and improvements.
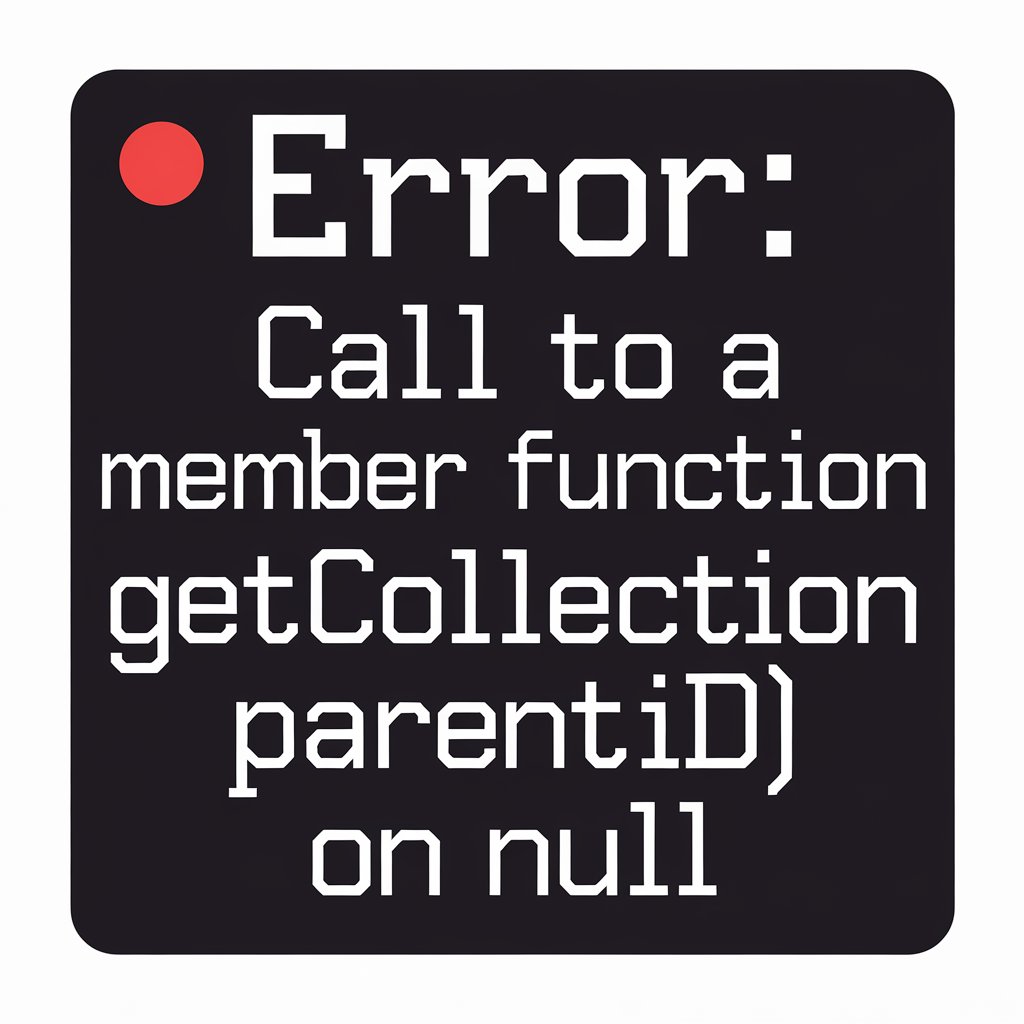
Example Use Case
Suppose you are working on a ConcreteCMS project and encounter this error while trying to fetch the parent page of a collection. The problem might be due to an invalid page ID. To resolve it, you could implement a solution like this:
$pageID = $_GET['pageID'] ?? null; // Fetch the page ID from the query parameter
if ($pageID) {
$collection = Page::getByID($pageID);
if ($collection) {
$parentID = $collection->getCollectionParentID();
echo "Parent Page ID: $parentID";
} else {
echo "Page not found.";
}
} else {
echo "Invalid or missing page ID.";
}
In this example, we validate the page ID before fetching the collection and check if the object exists before accessing its method. This ensures that the application does not crash due to null objects.
Also read: Raterpoint: A Comprehensive Insight
Conclusion
The “Call to a member function getCollectionParentID() on null” error can be frustrating, but it is entirely manageable with proper debugging and preventive coding practices. By understanding the root causes and applying the solutions outlined above, you can resolve this error effectively and build more robust applications. Always prioritize error handling and validation to ensure your code runs smoothly in all scenarios.